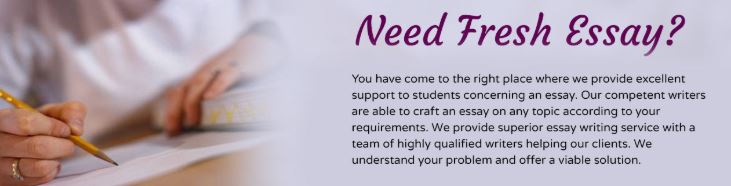
CSSE2010 / CSSE7201 Project, Semester 1, 2020 1The University of QueenslandSchool of Information Technology and Electrical EngineeringSemester 1, 2020CSSE2010 / CSSE7201AVR PROGRAMMING PROJECTDue: 12noon Friday 12 June 2020Weighting: 20% (100 marks)Specification update 1 June 2020. Changes shown in red.ObjectiveAs part of the assessment for this course, you are required to undertake a project which will test youagainst some of the more practical learning objectives of the course. The project will enable you todemonstrate your understanding of• C programming• C programming for the AVR• The Atmel Studio environment.You are required to modify a program in order to implement additional features. The program is asimple version of the game Space Impact. The AVR ATmega324A microcontroller runs the programand receives input from a number of sources and outputs a display to a LED matrix display board,with additional information being output to a serial terminal and, to be implemented as part of thisproject, a seven segment display and other LEDs.The version of Space Impact provided to you will implement basic functionality – the backgroundscrolls left to right and aliens appear and move but the player can only move in one direction (up) andthe game ends immediately when the player dies once. You can add features such as scoring, movingin other directions, multiple lives, sound effects, etc. The different features have different levels ofdifficulty and will be worth different numbers of marks.Don’t Panic!You have been provided with over 2700 lines of code to start with. Whilst this code may seemconfusing, you don’t need to understand all of it. The code provided does a lot of the hard work foryou, e.g., interacting with the serial port and the LED display. To start with, you should read theheader (.h) files provided along with project.c, player.c, projectile.c and alien.c. You may need tolook at the AVR C Library documentation to understand some of the functions used. A video will beprovided to introduce the project.Academic Merit, Plagiarism, Collusion and Other MisconductYou should read and understand the statement on academic merit, plagiarism, collusion and othermisconduct contained within the course profile and the document referenced in that course profile.You must not show your code to or share your code with any other student under anycircumstances. You must not post your code to public discussion forums or save your code inpublicly accessible repositories. You must not look at or copy code from any other student. Allsubmitted files will be subject to electronic plagiarism detection and misconduct proceedingswill be instituted against students where plagiarism or collusion is suspected. The electronicplagiarism detection can detect similarities in code structure even if comments, variable names,formatting etc. are modified. If you copy code, you will be caught.CSSE2010 / CSSE7201 Project, Semester 1, 2020 2Grading NoteAs described in the course profile, if you do not score at least 15% on this project (before any latepenalty) then your course grade will be capped at a 3 (i.e. you will fail the course). If you do notobtain at least 50% on this project (before any late penalty), then your course grade will be capped ata 5. Your project mark (after any late penalty) will count 20% towards your final course grade.Resubmissions prior to grade finalization are possible to meet the 15% requirement in order to passthe course, but a late penalty will be applied to the mark for final grade calculation purposes.Program DescriptionThe program you will be provided with has several C files which contain groups of related functions.The files provided are described below. The corresponding .h files (except for project.c) list thefunctions that are intended to be accessible from other files. You may modify any of the providedfiles. You may add additional files if desired. You must submit ALL files used to build your project,even if you have not modified some provided files. Many files make assumptions about which AVRports are used to connect to various IO devices. You should not change these.• project.c – this is the main file that contains the event loop and examples of how time-basedevents are implemented. You should read and understand this file.• game_position.h/game_position.c – contains an explanation of how positions arerepresented in the game, some useful macro definitions, and some functions to calculateneighbouring positions.• player.h/player.c – contains code related to the player in the game (e.g. player movementand display). You should read these files and understand the representation used for the player.You will need to modify this file to add required functionality.• alien.h/alien.c – contains code related to the aliens. You should read at least the header file(alien.h) and you may need to read/modify the .c file for some of the more advancedfunctionality.• projectile.h/projectile.c – contains code related to the projectiles fired by the player. Youshould read at least the header file (projectile.h) and you may need to read/modify the .c filefor some of the more advanced functionality.• game_background.h/game_background.c – contains code related to the scrolling gamebackground. You should read at least the header file (game_background.h) and you may needto read/modify the .c file for some of the more advanced functionality.• buttons.h/buttons.c – contains the code which deals with the IO board push buttons. It setsup pin change interrupts on those pins and records rising edges (buttons being pushed).• ledmatrix.h/ledmatrix.c – contains functions which give easier access to the servicesprovided by the LED matrix. It makes use of the SPI routines implemented in spi.c• pixel_colour.h – contains definitions of some useful LED matrix colours• score.h/score.c – contains code for keeping track of and adding to the score. This module isnot used in the provided code.• scrolling_char_display.h/scrolling_char_display.c – contains code which provides ascrolling message display on the LED matrix board.• timer0.h/timer0.c – contains code that sets up a timer that is used to generate an interruptevery millisecond and keep track of a global time value.• serialio.h/serialio.c – contains code that is responsible for handling serial input and outputusing interrupts. It also maps the C standard IO routines (e.g. printf() and fgetc()) to use theserial interface so you are able to use printf() etc for debugging purposes if you wish. Youshould not need to look in this file, but you may be interested in how it works and the buffersizes used for input and output (and what happens when the buffers fill up).• spi.h/spi.c – contains code that encapsulates all SPI communication. Note that by default, allSPI communication uses busy waiting – the “send” routine returns only when the data is sent.CSSE2010 / CSSE7201 Project, Semester 1, 2020 3If you need the CPU cycles for other activities, you may wish to consider converting this tointerrupt based IO, similar to the way that serial IO is handled.• terminalio.h/terminalio.c – this encapsulates the sending of various escape sequences whichenable some control over terminal appearance and text placement – you can call thesefunctions (declared in terminalio.h) instead of remembering various escape sequences.Additional information about terminal IO will be provided on the course Blackboard site.• line_drawing_characters.h – contains definitions of some Unicode line drawing charactersthat may be useful for terminal output. The definitions are not used in the supplied code.Game ElementsThe figure below illustrates the terminology used in the game and how the various game elements areshown on the LED matrix display.The expected behaviour of each of the elements is as follows:• The game background scrolls from right to left. The pattern repeats each 32 columns.• The player (2 x 1 pixels) can move anywhere on the game field but dies if it moves into thebackground or an alien (or if the background or an alien moves into the player). The playerwill be shown in light yellow if it dies.• The player can fire projectiles. Projectiles (single pixels) move from left to right. Projectilesthat run into the background or the player are removed from the display.• Aliens (2 x 2 pixels) start from the right-hand side and randomly move up, down and to theleft. They cannot move into another alien or the background. Aliens die and are removed fromthe display when hit by 10 projectiles; or when unable to move when pushed by a piece ofscrolling background; or if they fall off the left-hand side of the display. Up to 5 aliens can beon the display at any one time. (If a projectile hits an alien then the hit pixel will change colourto light orange – until the alien next moves.)Initial OperationThe provided program responds to the following inputs:• Rising edge on the button connected to pin B3 (causes the player to fire a projectile);• Serial input space character (causes the player to fire a projectile);• Rising edge on the button connected to pin B1 (causes the player to move up); and• Serial input escape sequence corresponding to the cursor-up key (causes the player to moveup).Code is present to detect the following, but no actions are taken on these inputs:• Rising edge on the button connected to B0 (intended to be move player down);CSSE2010 / CSSE7201 Project, Semester 1, 2020 4• Rising edge on the button connected to B2 (intended to be game speed toggle);• Serial input escape sequence corresponding to the cursor-down key (also move down);• Serial input escape sequence corresponding to the cursor-left key (intended to be move playerleft);• Serial input escape sequence corresponding to the cursor-right key (intended to be moveplayer right);• Serial input characters ‘p’ and ‘P’ (intended to be the pause/unpause key)Program FeaturesMarks will be awarded for features as described below. Part marks will be awarded if part of thespecified functionality is met. Marks are awarded only on demonstrated functionality in the finalsubmission – no marks are awarded for attempting to implement the functionality, no matter howmuch effort has gone into it, unless the feature can be demonstrated. You may implement higherlevel features without implementing all lower level features if you like (subject to prerequisiterequirements). The number of marks is not an indication of difficulty. It is much easier to earn thefirst 50% of marks than the second 50%. Marks for each feature are shown on the marksheet on thelast page.You may modify any of the code provided and use any of the code from learning lab sessions and/orposted on the course Blackboard site. For some of the easier features, the description below tells youwhich code to modify or there may be comments in the code which help you.Minimum Performance (Level 0 – Pass/Fail)Your program must have at least the features present in the code supplied to you, i.e., it must buildand run and allow the background to scroll, the player to be moved and a projectile to be fired. Nomarks can be earned for other features unless this requirement is met, i.e., your project mark will bezero.Splash Screen (Level 1)Modify the program so that when it starts (i.e. the AVR microcontroller is reset) it scrolls a messageto the LED display that includes your student number. You should also change the message output tothe serial terminal to include your name and student number. Do this by modifying the functionsplash_screen() in file project.c.Move Player Down (Level 1)The provided program will only move the player up. You must complete themove_player_down() function in file player.c. This function must not allow the player to moveoff the game area. The function should also check whether the move causes the player to die. If theplayer moves in to a projectile, the projectile must be removed.Move Player Left and Right (Level 1)You must complete the move_player_left() and move_player_right()functions in fileplayer.c. The functions must not allow the player to move off the game area. The function shouldalso check whether the move causes the player to die. If the player moves in to a projectile, theprojectile must be removed.Scoring #1 (Level 1)Add a scoring method to the program so that 1 is added to the score each time a projectile hits analien or an alien hits a projectile. You should make use of the function add_to_score(uint16_tvalue) declared in score.h. You should call this function (with an appropriate argument) from anyother function where you want to increase the score. If a .c file does not already include score.h, youmay need to #include it. You must also add code to display the score to the serial terminal in a fixedCSSE2010 / CSSE7201 Project, Semester 1, 2020 5position and update the score display only when it changes. The score must be displayed in a blockthat looks like this (with identical column alignment):Score0The displayed score must be right-aligned, i.e. the right-most digit in the score must be in a fixedposition – in line with the right-most character in the title (“Score”). (Hint – consider the formatspecifiers for printf().) A score of 0 must be displayed when the game starts. The score mustremain displayed on game-over (until a new game commences when the score should be reset to 0).High Score (Level 1)Prerequisite: Scoring #1 is implementedYou must also keep track of the high score (initially 0 on power-on) and update it whenever there isa new high score – including during game play if the current game has the highest score. You mustalso add code to display the high score to the serial terminal in a fixed position immediately belowthe current score. The scores must be displayed in a block that looks like this (with right alignmentof all elements):Score0High Score11240The high score display must be updated immediately when the high score changes and only when itchanges. A high-score of 0 must be displayed for the first game after power-on (or hardware reset).For later games, the current high score should be shown. The score (and high-score) must remaindisplayed on game-over (until a new game commences when the score should be reset to 0 but thehigh-score should continue to be displayed).Scoring #2 (Level 1)Prerequisite: Scoring #1 is implementedIn addition to the scoring described in Scoring #1, 5 points should be added to the score when aprojectile hit causes the alien to die. (You can choose to also add 1 point for the projectile hitting thealien as per Scoring #1, i.e. add 6 points total; or you can just add 5 points total.) The score must alsobe displayed on the seven-segment display. For scores from 0 to 9 inclusive the left seven segmentdigit should be blank. If the score is greater than 99 then make a reasonable assumption about whatshould be displayed. (This may not be tested.) No display flickering or ghosting1 should be apparent.Both digits (when displayed) should be of equal brightness. The score can be maintained on the sevensegment display when the game is over, or the display can be blanked. (Note that the score mustremain displayed on the terminal display – as per Scoring #1 above.)New Game (Level 1)Add a feature so that if the ‘n’ or ‘N’ key is pressed on the serial terminal, a new game is started (atany time, including if the splash screen is showing or if the current game is in progress or if a “gameover” screen is showing or …). Game play must commence immediately after the key is pressed, i.e.,it must not be necessary to press another button/key for play to start. All aspects of the game shouldbe reset to the starting point, except any high scores (if implemented). This feature must beimplemented in such a way that the game can be restarted an unlimited number of times without thegame crashing. Note that if the high score functionality is implemented and the current score is thehigh score when ‘n’ or ‘N’ is pressed then this score must be recorded as a high score.1 Ghosting is when the left digit value can be seen faintly on the right digit, or vice-versa.CSSE2010 / CSSE7201 Project, Semester 1, 2020 6If multiple levels are implemented (see next item), you can (but don’t have to) wait until after the“LEVEL UP” message finishes scrolling before handling any ‘n’ or ‘N’ pressed while the message isscrolling. It is unlikely that this will be tested.Multiple Levels (Level 2)Prerequisite: Scoring #1, Movement in all directionsModify the program to support multiple levels of game play. When the score reaches 100 (and eachmultiple of 100 – though this is unlikely to be tested) then play should stop and the message “LEVELUP” should scroll across the LED matrix before play immediately recommences on the next level.The second level must use a different scrolling background pattern with a different colour (butsubsequent levels can just alternate between patterns). The level number (starting at 1) must be shownon the terminal display immediately below the score (or high score if implemented) as shown:Score120High Score240Level2The level number must be shown on the terminal display whenever the score is shown (but must onlybe updated when the level changes). In order to achieve marks for this feature, a reasonable gameplayer (a tutor) must be able to reach a level-up within 90 seconds of commencing game play.The player position can be either reset or preserved (if it doesn’t clash with the background) when anew level is started. You can reset (i.e. remove) or preserve the positions of projectiles and aliens (butagain, if you preserve the positions they must not clash with the new background).Multiple Lives – Health Bar (Level 2)Modify the program to support multiple lives, i.e., the player starts with 4 lives and must “die” fourtimes before the game is over. When a life is lost, the game display should show this for about twoseconds (i.e. display is frozen (i.e. movement stops) with the dead player showing) before playimmediately resumes2 with a reset game field. (Note that the score, if implemented, does not reset.The reset game field should be on the same level – if multiple levels are implemented. You can resetthe background scroll position or leave it the same – your choice.) The number of lives remainingmust be indicated using 4 LEDs as a “health bar” (L5, L4, L3 and L2 on the IO Board – which aregreen, red, orange and green respectively). When one life is lost, a green LED should be switchedoff. When the second is lost, the other green LED is switched off. When the third is lost, the orangeLED is switched off (leaving only the red). When the last life is lost, all four LEDs should be off. Thenumber of lives remaining (4 down to 1) must also be shown on the terminal display in a fixedposition. (When all lives are lost, the terminal display should show 0 lives remaining, or the numberof lives remaining should not be shown at all.) Note that the connection to the 4 LEDs must be ableto be made with a single 4-wire jumper cable from 4 adjacent IO pins on the AVR microcontrollerboard. No LED flickering should be apparent.If multiple levels are implemented, you can add back a life if you wish when a new level is reached– provided you do not exceed 4 lives at any point.Auto-repeat (Level 2)Prerequisite: Moving player down.Add auto-repeat support to the push buttons (B0, B1 and B3) which move the player and fireprojectiles – i.e., if one or more push buttons are held down then, after a short delay, they should actas if they are being repeatedly pressed. The auto-repeat behaviour on each button should be2 i.e. no button or key presses are required to resume play with the next lifeCSSE2010 / CSSE7201 Project, Semester 1, 2020 7independent, e.g. if button 1 is in auto-repeat mode (i.e. you’re holding it down) and you also pressand hold button 3 then button 1 should continue in auto-repeat mode and, after whatever delay youhave set, button 3 would enter this mode also. (If the buttons for both moving up and moving downare held down then the player should not move3. In cases where an up/down keyboard character isalso received while both buttons are held down, then you can choose to do whatever you like, e.g.ignore it or move that way – just choose something sensible – the game shouldn’t crash and the playershouldn’t suddenly jump a large distance.) For cases where one up/down button is held down andconflicting keyboard presses are received, you can again choose to do whatever you like provided itis sensible. In this case, the player may will move up and down between two positions, but it doesn’thave to.Game Speed Toggle (Level 2)Prerequisite: Scoring #1The provided program scrolls the background at a speed of one pixel every 600ms, moves an alienevery 400ms and moves projectiles every 300ms. Modify the program so that pressing button B2toggles (i.e. swaps) between game play at this speed and a double speed mode where these times (andno others) are halved. During double speed mode play the scores earned for projectile hits must bedoubled, including points for killing aliens. When double speed mode is active, this must be indicatedby a message on the terminal display and the rightmost decimal point on the seven segment displaymust be illuminated. Double speed mode continues until the button is pressed again, or the game isover, e.g. it stays active if the player starts a new level or loses a life (other than the final life).Game Pause (Level 2)Modify the program so that if the ‘p’ or ‘P’ key on the serial terminal is pressed then the game willpause. When the button is pressed again, the game recommences. (The check for this key press isimplemented in the supplied code, but does nothing.) All button presses (B0 to B3) and keyboardinput (other than the ‘p/P’ key and those keys mentioned below) should be discarded whilst the gameis paused, i.e. no player movement or projectile firing happens after unpausing the game unless suchbuttons/keys are pressed after that time. The game movement rates must be unaffected – e.g. if thepause happens 200ms before the background is due to scroll, then the background must not scrolluntil 200ms after the game is resumed, not immediately upon resume.While the game is paused, a message indicating this must be displayed on the terminal display. (Thescore and other data normally displayed during game play must remain displayed.) If Scoring #2 isimplemented then the score must remain displayed on the seven segment display.If “new game” functionality is implemented (see above) then the ‘n’ and ‘N’ key presses are notignored during game pause – a new game should be started immediately.If the “EEPROM save game” functionality is implemented (see below) then the ‘s’ and ‘S’ key pressesare not ignored – the current game state is saved. Similarly, the ‘o’ and ‘O’ key presses are not ignored– the saved game state (if available) is restored immediately.Sound Effects (Level 3)Add sound effects to the program which are to be output using the piezo buzzer. You must specifywhich AVR pin the piezo buzzer is connected to. (The buzzer will be connected between there andground.) Different sound effects (tones or combinations or tones) must be implemented for at leastfour events. (At least two of these must be sequences of three or more tones for full marks.) Choosefour of:• Projectile being fired3 It may move up and then down (or vice versa) initially based on the first press of each button but there should be no auto-repeat ifboth are held down. There should be auto-repeat functionality if both a movement and the firing button are held down together.CSSE2010 / CSSE7201 Project, Semester 1, 2020 8• Player moving• Projectile hitting alien• Alien dying• Player dying (game over or loss of life)• Game start up (this could be when the splash screen is showing or commences being shownOR it could be when game play starts on commencement of a new game – including the firstgame)• Constant background tuneDo not make the tones too annoying! Switch 7 on the IOboard must be used to toggle sound on andoff (1 is on, 0 is off). Your feature summary form must indicate which events have different soundeffects. Sounds must be tones (not clicks) in the range 20Hz to 5kHz. Sound effects must not interferewith game play, e.g. the speed of play should be the same whether sound effects are on or off. Soundmust turn off if the game is paused.EEPROM Game Storage (Level 3)Prerequisite: Scoring #1Implement storage of the complete game state in EEPROM. If the “s” or “S” key (for “Save”) is sentfrom the serial terminal then the whole game state should be saved – and a message indicating thisshould be output to the terminal. If the “o” or “O” key (for “Open”) is later sent (possibly after theboard is powered-off and then back on), then the saved game should be retrieved and play shouldcontinue on in that game. You must handle the situation of the EEPROM initially containing dataother than that written by your program. You may need to use a “signature” value to indicate whetheryour program has initialized the EEPROM for use – it should not be possible to “open” a saved gameif none was saved from your program. When your game starts (i.e. after/during the power-up splashscreen) it should indicate on the terminal display whether a saved game exists in EEPROM or not.The game state includes the following– the location of the player– the location of all aliens– the location of all projectiles– the scroll position of the scrolling background– the current score– the high score (if implemented)4– the number of lives remaining (if multiple lives are implemented)– the current level (if multiple game levels are implemented)Game state does not include– whether the game is paused or not – the game will resume in an unpaused state when “opened”– whether double speed mode is active or not – the game will resume at the normal (slower)speed– the timing of movement, i.e. how much time to the next movement of any game element –though you can record this if you wish (for no additional marks).The key presses (s/S/o/O) must be detected when the game is playing or when the game is paused.You may also detect them at other times (e.g. detecting “o” or “O” on splash screen or game over)but you do not have to.4 Note that even if a higher score has been achieved since the game was saved, the high score that was saved to EEPROM will be theone that is restored.CSSE2010 / CSSE7201 Project, Semester 1, 2020 9Joystick (Level 3)Prerequisite: Movement in all directionsAdd support to use the joystick to move the player in all directions. If the joystick is moved in onedirection then the player must move in that direction, provided that move is still within the game field.Auto-repeat support must be implemented – if the joystick is held in one position then, after a shortdelay, the code should act as if the joystick was repeatedly moved in that direction. Note that diagonalmovement support must be supported – i.e. the player must move diagonally if possible – or in oneof the two associated directions if only that direction is possible5.Be aware that different joysticks may have slightly different min/max/resting output voltages and youshould allow for a range of values in your implementation – your code will be marked with a differentjoystick to the one you test with.Game Display on Terminal Screen (Level 3)Display a copy of the LED matrix display on the serial terminal using block graphics (e.g. reversevideo) and characters of various colours – possibly different colours to those used on the LED matrix.This should allow the game to be played either by looking at the LED matrix or at the serial terminal.(The serial terminal display must keep up with the LED matrix display, i.e. no apparent differencebetween the displays.) The baud rate must remain at 38400. You can assume that the terminal displaywill be at least 80 columns in width and 24 rows in height (i.e. the default size in PuTTY). You willneed to draw an appropriate border to indicate the game field. The speed of game play must not beadversely affected by the presence of this feature.Visual Effects on the LED display (Level 3)Implement visual effects on the LED display – i.e. multi-pixel animations in response to at least twoevents, e.g. an explosion effect when a projectile hits an alien. Select two of the following events:• projectile hitting alien (or vice versa) – but which doesn’t cause the alien to die• alien dying after projectile hit• player losing a lifeJust changing colours on existing game elements (e.g. an alien) is not sufficient. The animation shouldextend to pixels beyond those used for the game elements – but not interfere with the display afterthe animation is finished. Note that scrolling a message on the LED matrix display does not count asa multi-pixel animation. The two animations should be different – e.g. it is not appropriate to use thesame explosion animation when a projectile hits an alien as is used for the player losing a life. Visualeffects do NOT need to be shown on the terminal screen (if Game Display on Terminal Screen isimplemented) but can be if you wish.5 For example, if the joystick is moved diagonally to the upper left, but the player is already at the top edge of the game field then theplayer should just move to the left if that is possible.CSSE2010 / CSSE7201 Project, Semester 1, 2020 10Assessment of Program ImprovementsThe program improvements will be worth the number of marks shown on the mark sheet. You willbe awarded marks for each feature up to the maximum mark for that feature. Part marks will beawarded for a feature if only some part of the feature has been implemented or if there arebugs/problems with your implementation (which may include issues such as incorrect data directionregisters). Your additions to the game must not negatively impact the playability or visual appearanceof the game. Note also that the features you implement must appropriately work together, for example,if you implement game pausing then sound effects should pause as well.Features are shown grouped in their levels of approximate difficulty (level 1, level 2, and level 3).Some degree of choice exists at level 3, but the number of marks to be awarded here is capped, i.e.,you can’t gain more than 20 marks for advanced features even if you successfully add all thesuggested advanced features. You can’t receive more than 100 marks for the project as a whole.Submission DetailsThe due date for the project is 12noon Friday 12 June 2020. The project must be submitted viaBlackboard. You must electronically submit a single .zip file containing ONLY the following:• All of the C source files (.c and .h) necessary to build the project (including any that wereprovided to you – even if you haven’t changed them);• Your final .hex file6 (suitable for downloading to the ATmega324A AVR microcontrollerprogram memory); and• A PDF feature summary form (see below).Do not submit .rar or other archive formats – the single file you submit must be a zip format file. Allfiles must be at the top level within the zip file – do not use folders/directories or other zip/rar filesinside the zip file.If you make more than one submission, each submission must be complete – the single zip file mustcontain the feature summary form and the hex file and all source files needed to build your work. Wewill only mark your last submission and we will consider your submission time (for late penaltypurposes) to be the time of submission of your last submission.The feature summary form is on the last page of this document. A separate electronically-fillablePDF form will be provided to you also. This form can be used to specify which features you haveimplemented and how to connect the ATmega324A to peripherals so that your work can be marked.If you have not specified that you have implemented a particular feature, we will not test for it. Failureto submit the feature summary with your files may mean some of your features are missed duringmarking (and we will NOT remark your submission). You can electronically complete this form oryou can print, complete and scan the form. Whichever method you choose, you must submit a PDFfile with your other files.Incomplete or Invalid CodeIf your submission is missing files (i.e. won’t compile and/or link due to missing files) then we willsubstitute the original files as provided to you. No penalty will apply for this, but obviously nochanges you made to missing files will be considered in marking.If your submission does not compile and/or link in Atmel Studio 7 for other reasons, then the markerwill make reasonable attempts to get your code to compile and link by fixing a small number of simple6 The .hex file can be found in the “Debug” folder within your Atmel Studio project.CSSE2010 / CSSE7201 Project, Semester 1, 2020 11syntax errors and/or commenting out code which does not compile. A penalty of between 10% and50% of your mark will apply depending on the number of corrections required. If it is notpossible for the marker to get your submission to compile and/or link by these methods then you willreceive 0 for the project (and will have to resubmit if you wish to have a chance of passing the course).A minimum 10% penalty will apply, even if only one character needs to be fixed.Compilation WarningsIf there are compilation warnings when building your code (in Atmel Studio 7, with default compilerwarning options) then a mark deduction will apply – 1 mark penalty per warning up to a maximumof 10 marks. To check for warnings, rebuild ALL of your source code (choose “Rebuild Solution”from the “Build” menu in Atmel Studio) and check for warnings in the “Error List” tab.Late SubmissionsLate submission will result in a penalty of 10% plus 10% per calendar day or part thereof, i.e.a submission less than one day late (i.e. submitted by 12noon Saturday 13 June 2020) will bepenalised 20%, less than two days late 30% and so on. (The penalty is a percentage of the mark youearn (after any of the other penalties described above), not of the total available marks.) Requests forextensions should be made via the process described in the course profile (before the due date) andbe accompanied by appropriate documentary evidence of extenuating circumstances (e.g. personalstatement or medical certificate). The application of any late penalty will be based on your latestsubmission time.Notification of ResultsStudents will be notified of their results via Blackboard’s “My Grades” when marking is complete.The University of Queensland – School of Information Technology and Electrical EngineeringSemester 1, 2020 – CSSE2010 / CSSE7201 Project – Feature Summary
Student Number
Family Name
Given Names
An electronic version of this form will be provided. You must complete the form and include it (as a PDF) in yoursubmission. You must specify which IO devices you’ve used and how they are connected to your ATmega324A.
Port
Pin 7
Pin 6
Pin 5
Pin 4
Pin 3
Pin 2
Pin 1
Pin 0
A
B
SPI connection to LED matrix (B7 to SCK etc.)
Button B3
Button B2
Button B1
Button B0
C
D
Serial RX
Serial TX
Baud rate: 38400
Feature
ü ifattempted
Comment(Anything you want the marker to consider or know?)
Mark
Splash screen
/4
Move PlayerDown
/5
Move Player Leftand Right
/5
Scoring #1
/10
High Score
/10
Scoring #2
/10
New Game
/10
/54
Multiple Levels
/6
Multiple Lives –Health Bar
/6
Auto-repeat
/6
Game SpeedToggle
/6
Game Pause
/6
/30
Sound Effects
/5
EEPROM GameStorage
/5
Joystick
/5
Game Display onTerminal Screen
/5
Visual Effects onLED display
/5
/20 max
Total: (out of 100, max 100)Penalties: (code compilation, incorrect submission files, etc. Does not include late penalty)Final Mark: (excluding any late penalty which will be calculated separately)
[Button id=”1″]
Quality and affordable writing services. Our papers are written to meet your needs, in a personalized manner. You can order essays, annotated bibliography, discussion, research papers, reaction paper, article critique, coursework, projects, case study, term papers, movie review, research proposal, capstone project, speech/presentation, book report/review, and more.
Need Help? Click On The Order Now Button For Help
What Students Are Saying About Us
.......... Customer ID: 12*** | Rating: ⭐⭐⭐⭐⭐"Honestly, I was afraid to send my paper to you, but splendidwritings.com proved they are a trustworthy service. My essay was done in less than a day, and I received a brilliant piece. I didn’t even believe it was my essay at first 🙂 Great job, thank you!"
.......... Customer ID: 14***| Rating: ⭐⭐⭐⭐⭐
"The company has some nice prices and good content. I ordered a term paper here and got a very good one. I'll keep ordering from this website."