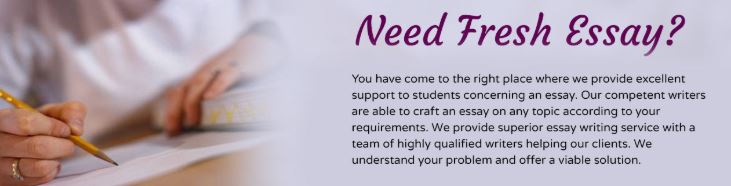
FACULTY OF LAW & BUSINESSPeter Faber Business SchoolITEC313: Object Oriented Programming, Semester 1, 2020Assessment 1 [Weight 50%]Page 11. Your first task is to design and create a RiverCrossing class to represent the river crossing with theappropriate attributes and methods. Assume river flows west to east (left to right of the page),farmer moves north (straight up) and requires to transport items from the South Bank to NorthBank of the river. Design class so it can be re-used in Part 2 of the assignment, the GUI version ofRiver Crossing.
(a)(b)
Write the RiverCrossing constructor.Use a boolean (or integer) array elements as place holders for the Farmer’s items (boat,cabbage, goat, wolf). False value to represent an item located on the South Bank and Trueon the North Bank of river.Write a row() method to transport an item from one Bank to another Bank.Write get() methods to return string with inventory (a list of all items, including the boat)located on the North Bank and another get() method for the South Bank.Write other methods as required.
(c)(d)
(e)
2. Write a Command Line Interface class with main method to allow the user to play the game. Asample of a dialogue between the computer and the user (farmer) trying to cross from the southBank to the north Bank:cabbage, wolfRiver Crossing, Game Programming AssignmentDue date: 11:59 pm on Friday the 15th of May, 2020 (Group of 2 max)You are to work with a partner, 2 students per group is the maximum. Group must submit for bothstudents, only one copy of the assignment. Submission details are at the end of this document.The assignment is composed of two parts.Problem DescriptionOne day a farmer goes to the market, taking with him a cabbage and a goat. On the way he captured awolf, he’s a mighty farmer, and took it with him to the market. They came to a river. And on the Bank,there’s a boat. Now, the problem is that the boat just big enough to carry the farmer and one of the others.If the farmer leaves the wolf and the goat together, in his absence, the wolf would make a meal of the goat.Neither, could be leave the goat and the cabbage alone: the goat would eat the cabbage. Being used tohardship, the farmer was quite prepared to make several trips back and forth, just so long as he couldeventually bring them all to the other side and went on to the market. How should he arrange the trips?North Bank: boat, goatSouth Bank:~~~~~~~~~~~~~~~~~~~~~~~~~~~~~River~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~Figure 1. CLI version of the river crossingPart 1 RequirementsYour task as a software developer is to write a Command Line Interface (CLI) applicationRiverCrossingCLI simulating the river crossing. The farmer (player) can then work out the steps requiredto safely move all items to the other side of the river. In Part 1 you will need to do two tasks:South Bank itemsNorth Bank itemsITEC313 Object Oriented ProgrammingPage 2Sample 1Welcome to the CLI version of the River Crossing Game.Farmer crossing a river has boat, cabbage, goat and wolf.Boat can carry two items at the time and cannot leavethe cabbage alone with the goat or the goat with the wolf.North Bank:────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: boat, cabbage, goat, wolfYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 3North Bank: boat, goat────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: cabbage, wolfYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 1North Bank: goat────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: boat, cabbage, wolfYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 4North Bank: boat, goat, wolf────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: cabbageYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 3North Bank: wolf────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: boat, cabbage, goatYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 2North Bank: boat, cabbage, wolf────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: goatYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 1North Bank: cabbage, wolf────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: boat, goatYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 3North Bank: boat, cabbage, goat, wolf────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank:***** You won *****ITEC313 Object Oriented ProgrammingPage 3Sample 2Welcome to the CLI version of the River Crossing Game.Farmer crossing a river has boat, cabbage, goat and wolf.Boat can carry two items at the time and cannot leavethe cabbage alone with the goat or the goat with the wolf.North Bank:────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: boat, cabbage, goat, wolfYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 2North Bank: boat, cabbage────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: goat, wolfThe wolf ate the goat***** Game over *****Sample 3Welcome to the CLI version of the River Crossing Game.Farmer crossing a river has boat, cabbage, goat and wolf.Boat can carry two items at the time and cannot leavethe cabbage alone with the goat or the goat with the wolf.North Bank:────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: boat, cabbage, goat, wolfYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 3North Bank: boat, goat────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: cabbage, wolfYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)? 4North Bank: boat, goat────────────────────────────────────────~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~────────────────────────────────────────South Bank: cabbage, wolf***** I don’t see the wolf on the North BankYou have the following choices:1 Row across by yourself2 Take the cabbage with you3 Take the goat with you4 Take the wolf with you5 QuitWhat is your choice (1, 2, 3, 4 or 5)?ITEC313 Object Oriented ProgrammingPage 4The above dialogs are meant as a sample only. You are at liberty to design the game in any way you likeand you should. In particular, you should inform the user the consequences of a bad decision, i.e., thecabbage or the goat was eaten and the game is overA sample of an UML diagram shows the class diagram forRiverCrossing class.Instance attributes are all private;• Bank[ ] array contains boolean values representing position ofan item, south Bank false and true if its in the north Bank• item[ ] array comprise of strings “boat”, “cabbage”, “goat”,“wolf”• other attributes as necessaryRiverCrossing Methods• RiverCrossing( ) constructor sets all item to false (south Bank)• getItemStatus(int item) returns a String with location of item i• getNorthBank( ) return String with north Bank inventory• getSouthBank( ) return String with south Bank inventory• row(int item) transport the boat with the selected item. Farmer is allowed to take an empty boatacross the river. The method returns the following integer codes:0 the move successful1 all items transported to north Bank, player won11 the selected item is already across the river, error code12 the goat ate the cabbage, end of the game13 the wolf ate the goat, end of the game14 the goat ate the cabbage and wolf ate the goat, end of the game.Part 2Create a Windows GUI (JFrame) application for launching RiverCrossing game.Choose GUI components that reduce user errors and provide a feedback to the player. For example, thereis no need for a “boat” button because “row” button always moves the boat with or without an item.One simple GUI interface is shown in the Figure 2 below. Click the button “row” to immediately row theboat across or to transport the wolf select button “wolf” followed by click on the “row” button.
River Crossing
– Bank[] : Boolean– item[] : final String– other attributes
+ RiverCrossing( )+ getItemStatus(int) : String+ getNorthBank( ) : String+ getSouthBank( ) : String+ row(int) : int+ other methods
error codes
DisplayRiver
– other attributes
+ printRiver() : void+ other methods
//Create JFrame containerpublic class River
public static void main(String[] args)
//main method
JFrame frame = new JFrame(“River Crossinge”);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.getContentPane().add(new RiverGUI()); //create GUIframe.pack();frame.setVisible(true);//end of main method//end River classITEC313 Object Oriented ProgrammingPage 5Figure 2. Basic river crossing layout.Once again, this is only a sample interface to illustrate the idea of the game. You are free to choose yourown colour scheme and buttons. Movement can be achieved by clicking a button. Make use of layoutmanagers to design colourful and a well-organized game. Consider the following controls as a minimumrequirement:• one button to reset and start the game again,• a text field for the player’s name (a player may choose to leave it blank).• a text area to display a warning messages (and the past history), for example, “This is not a goodidea” or to inform the player that cabbage or the goat was eaten and game is lost. You can provideyour own appropriate messages.An advanced River crossing is shown in Figure 3. Full marks will be given to fully functional basic layoutbut additional marks may be given by better GUI as long it is fully functional.Figure 3. An example of advanced river crossing layout with button icons (pictures) and boat drawing.
Northresetgoat
Welcome to the River Crossing Game.Farmer wants to cross the river but hemust not leave the cabbage alonewith the goat or the goat with the wolf.You can– Row across by yourself
Southclear all cabbage goat wolfcabbagewolfrow
River
– Take the cabbage with you– Take the goat with you– Take the wolf with you
ITEC313 Object Oriented ProgrammingPage 6Submission Details and Marking SchemeIt is compulsory that you demonstrate your work to your lab instructor in week 11 or 12. Submission ofthe assignment with code and other deliverables (refer to ‘What you have to hand in’ section below) aredue on Friday the 15th of May 2020. Emailed submissions will not be accepted.The late assignments will be penalised 5% per day. However, if you have a good reason for notfinishing on time, you may apply for a Special Consideration.What do you have to hand in?An electronic copy of1. Readme.docx file with information on how to run your program. Include any extra informationabout your design and program that you wish the marker to know.2. Summary of tasks allocations—who did what?3. A word document with the evidence of trial runs of your program, i.e., screen printouts of the resultswhere you have tested all the features of your code.4. UML diagram for all classes in your program.5. Code for all the classes that has been compiled and are ready to run. (jGRASP will be used to runand test your application).6. A brief description of the class. At the start of each method, there should be a comment clearlydescribing what the method does.Each class should be fully documented commencing with a heading. In particular, the headingshould include your name, unit, assignment details, date written and a brief description of theclass. At the start of each method, there should be a comment clearly describing what the methoddoes. If you have a partner, their name and student number is should be clearly identified in theheading beside your nameITEC313 Object Oriented ProgrammingPage 7ITEC313 – AssignmentName………………………….. Name…………………………..ID No………………………. ID No……………………….Markers Guideline
AllocatedMarks
Markachieved
Appropriate design including UML class diagrams and description ofhow to solve the problem plus readme.docs file.Printout of extensive trial runs
10
class RiverCrossing( ) — design and implementation
10
Other classes for CLI application — game play design andimplementation.
10
class RiverGUI( )–Windows application — design and implementation.Any other classes.
10
Output & Functionality — program works and meets specification.Whole Program• Originality, user friendliness and presentation.• Appropriate commenting of code and readability of the code.
10
Total
50
3 Rubric
[Button id=”1″]
Quality and affordable writing services. Our papers are written to meet your needs, in a personalized manner. You can order essays, annotated bibliography, discussion, research papers, reaction paper, article critique, coursework, projects, case study, term papers, movie review, research proposal, capstone project, speech/presentation, book report/review, and more.
Need Help? Click On The Order Now Button For Help
What Students Are Saying About Us
.......... Customer ID: 12*** | Rating: ⭐⭐⭐⭐⭐"Honestly, I was afraid to send my paper to you, but splendidwritings.com proved they are a trustworthy service. My essay was done in less than a day, and I received a brilliant piece. I didn’t even believe it was my essay at first 🙂 Great job, thank you!"
.......... Customer ID: 14***| Rating: ⭐⭐⭐⭐⭐
"The company has some nice prices and good content. I ordered a term paper here and got a very good one. I'll keep ordering from this website."