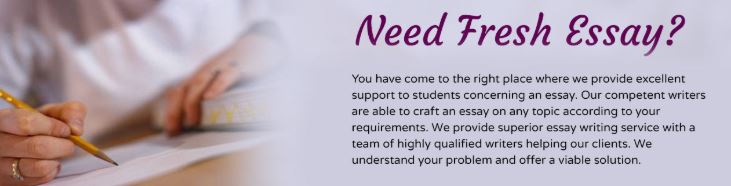
[ad_1]
About: Python polymorphism
Task: Numerical simulation of orbits
The task is to simulate orbits through numerical integration (described below). By swapping one object with another, you can change behavior without changing the other objects.
The system will consist of two components:
– Function objects. These represent the function to be integrated. In this exercise you will create two of these, one without air resistance and one with. By switching function objects, the same integrator can be used for both cases.
– The integrator. The integrator from this exercise is described in the next section.
Numerical integration:
Here is an introduction to how numerical integration is intended.
– You start at time 0 and with a start state that is a vector. For the example of trajectories, the vector is 4 long and contains the elements [x_position, y_position, x_speed, y_speed]. The integrator should be written as generally as possible and be able to take initial state vectors of lengths other than 4.
– Create a matrix (two-dimensional numpy array) where the first number (row) is what time step you are on and second number (column) is the position in the state vector of that time step.
– For each time step from start to finish:
Call the function with the state of the previous time step. The function returns a vector of the same length as the state vector representing how the state changes.
Take the state vector to the previous state. For each element, add the time step length multiplied by the corresponding value in the vector returned from the function.
Put this new state vector into the correct position in the matrix
– Return the matrix
Functions:
The functions in this exercise are represented by classes and should support the following interface (a single method):
– evaluate (time, state vector) -> change vector
The return value is after the arrow to the method.
THE ACTUAL TASKS:
Sub-tasks
a) Write a class for the numeric integrator described in the previous section. The constructor should take the length of the time steps as well as the end time as parameters. The integrator should support the method integrate(function object, initial state) -> matrix
b) Write a class for a basic ball path. The constructor must take into account how strong the gravity is in the constructor. The function is based on the state vector [x_position, y_position, x_speed, y_speed] and must return the change vector [x_speed, y_speed, 0, gravity]
c) Write a class for a sphere trajectory that takes into account air resistance. The constructor should take in how strong gravity is and a constant that states how much air resistance there is called c. The function uses the same state vector as the previous sub task and returns the change vector [x_speed, y_speed, – (c * x_speed * speed), gravity – (c * y_speed * speed)]. Speed is calculated as sqrt (x_speed ** 2 + y_speed ** 2)
d) Write a master program that creates the integrator, creates an object of each of the two functions, and runs the integrator on both. The main program should plot the result. The x and y coordinates of the result of the integration are the first and second columns of the matrix returned from the integrator. As a sample data for the plot you can use a. Gravity -9.81 (negative since positive y-direction is up) b. Time step 0.01 c. End time 0.45 d. Air resistance 0.5 e. Starting state [0,0,1,2]
“Looking for a Similar Assignment? Get Expert Help at an Amazing Discount!”
[ad_2]Source link
“Looking for a Similar Assignment? Get Expert Help at an Amazing Discount!”
What Students Are Saying About Us
.......... Customer ID: 12*** | Rating: ⭐⭐⭐⭐⭐"Honestly, I was afraid to send my paper to you, but splendidwritings.com proved they are a trustworthy service. My essay was done in less than a day, and I received a brilliant piece. I didn’t even believe it was my essay at first 🙂 Great job, thank you!"
.......... Customer ID: 14***| Rating: ⭐⭐⭐⭐⭐
"The company has some nice prices and good content. I ordered a term paper here and got a very good one. I'll keep ordering from this website."